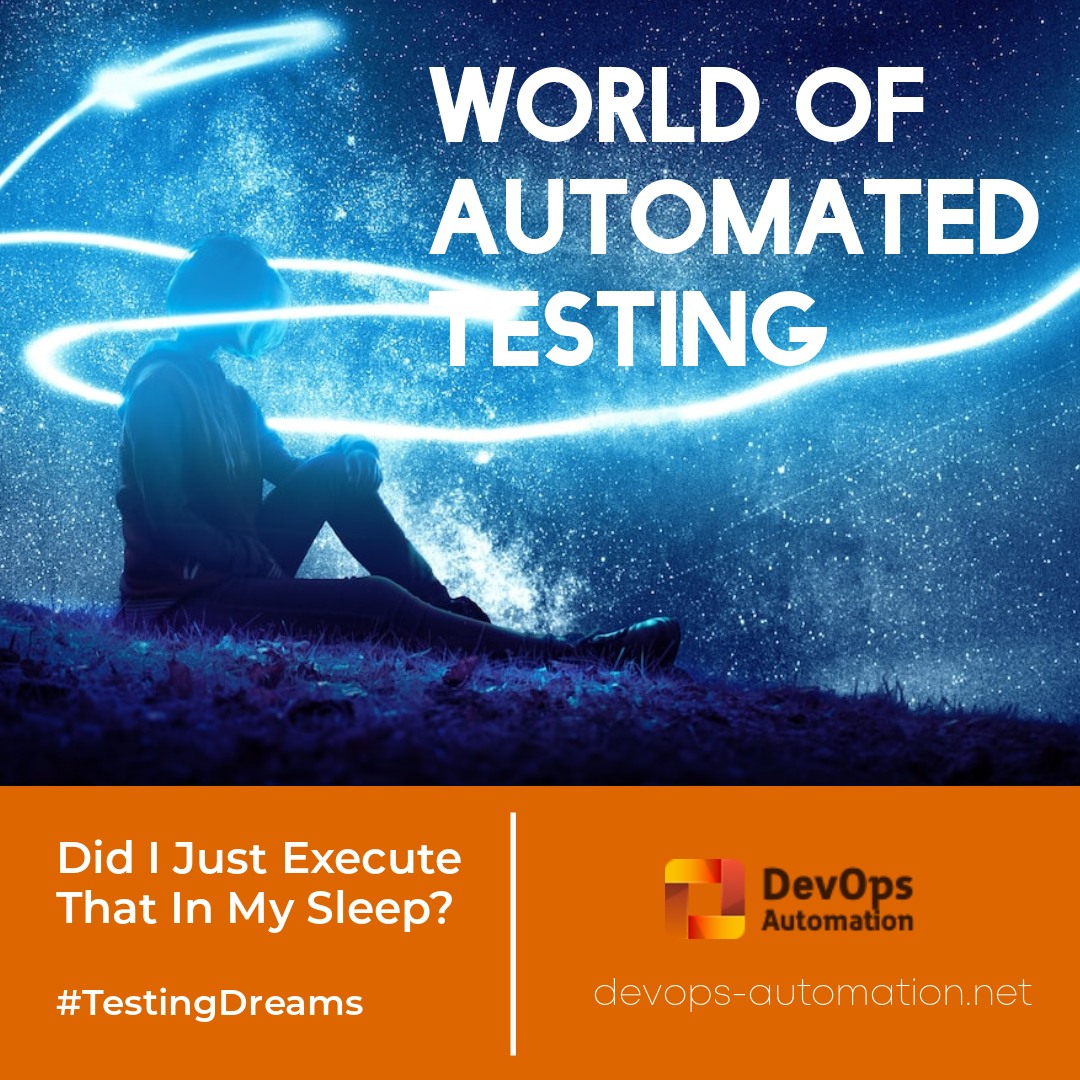
Selenium Automation Testing — Introduction
Unraveling the Magic of Automation Testing
Think about those times when you hit ‘run’ on a manual test, and a developer somewhere pulls their hair out in frustration. It’s like a secret plot to give them more grey hairs, right? Automation testing swoops in as the hero, catching those sneaky bugs before they become full-blown problems. It’s not just about speed; it’s a time machine that frees developers and testers to dream, innovate, and make the impossible possible. Learn automation testing and unleash this superpower in your software development journey.
Enter the Star of the Show: Selenium
Hold onto your coding hats because here comes Selenium — your trusty sidekick in the world of automation testing! This tool is like your tech-savvy buddy who’s got your back in testing. It’s not just popular; it’s a superstar in the testing arena, trusted by testers and developers worldwide.
Why the buzz, you ask? Well, Selenium is like an open-source rockstar that knows how to play well with different programming languages. Whether you’re fluent in Java, Python, or something in between, Selenium’s got your language covered. It’s the tool that makes testing not just efficient but actually enjoyable.
Ready to Dive In? Let’s Go!
So, get ready to roll up your sleeves and embark on this exciting journey into the world of Selenium. We’re here to make the complex simple, the mysterious clear, and the coding adventure downright exhilarating. It’s time to bring on the Selenium magic and unleash your testing superpowers!
Getting Started with Selenium
Downloading and Setting Up Selenium
You will need to grab selenium before you can launch the code. It’s as easy as downloading your favorite app — only better. Head to the Selenium website, choose your preferred language (Java or Python), and voilà! You’re set.
Embracing the Selenium WebDriver
WebDriver is a hero that’s interacting with the browsers. Imagine it as the driver of a fancy car — it takes you wherever you want to go. WebDriver will do this for you with Chrome, Firefox, Edge, and Safari. Import this into your code, and you’re ready to go.
Picking Your Programming Language
You’re an expert in Java or a Python wizard? It’s up to you. The choice is yours! Selenium supports those two languages, so stick to what gets your programmer’s heart beating. Don’t worry; we won’t judge you if you secretly hum Java tunes in your sleep. Might we be biased towards Java since we put up our course using Java? Well maybe :-) But hey! Python is an amazing scripting language too!
Selenium Components and Architecture
Selenium WebDriver
Mastering Selenium Java Testing Automation (w/ ChatGPT Boost) — Section 8 Slide
The WebDriver is where the real magic happens. Like the beloved wand, it makes the web elements dance for you. From buttons to dropdowns, you’ll use WebDriver’s locators to find and interact with them.
Exploring the Browser Landscape
WebDriver supports various browsers, just like your closet has different shoes for different occasions. Chrome, Firefox, Edge, Safari — pick your browser, and WebDriver’s got your back.
Creating WebDriver Instances
Creating a WebDriver instance is like summoning a ride-sharing service. You request a specific browser, and WebDriver provides a fancy car ready to whisk you away to your testing adventure.
Selenium Grid
Mastering Selenium Java Testing Automation (w/ ChatGPT Boost) — Section 10 Slide
The Selenium Grid is a planning tool for your testing parties. It lets you distribute your tests across multiple machines and browsers, saving you time and ensuring your app shines everywhere.
Writing Your First Selenium Test
Building Your Test Playground
Before you jump into testing, you need a safe space to play. Set up a project, import Selenium’s libraries, and you’re ready to start tinkering.
Navigating with Elegance
With WebDriver, navigating to a website is as easy as entering a URL. Just a few lines of code, and you’re there.
Finding Elements
Web elements are like hidden treasures on a webpage. With locators like IDs, Names, XPaths, and CSS Selectors, you’ll become a pro at finding them in no time.
WebElements Interaction
Clicking buttons and typing into text fields will make web elements bust a move with WebDriver’s methods. It’s Selenium coding choreography at its finest.
Writing Your Selenium Test (contd.)
Create Your Project
First things first, create a new project in your favorite Java IDE. We are big fans of IntelliJ IDEA IDE. I will share with you the steps for setting up a Maven project for automation testing using IntelliJ.
Step 1 — Create a new project
Step 2 — Name your project and select an archetype
Step 3 — Select JDK version and name your artifact
Once your project is set up, you must import the Selenium libraries to start using its magic. Here’s how:
import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver;
Navigating Using WebDriver
Time to make the browser dance to your tune! Here’s a snippet of Java code to open a webpage using the Chrome browser:
// set the path to the ChromeDriver executable System.setProperty("webdriver.chrome.driver", "path/to/chromedriver"); // initialize the ChromeDriver instance WebDriver driver = new ChromeDriver(); // open a webpage driver.get("https://www.devops-automation.net"); // close the browser when you're done driver.quit();
Finding Elements
Time to make the browser dance to your tune! Here’s a snippet of Java code to open a webpage using the Chrome browser:
// finding an element by its ID WebElement searchBox = driver.findElement(By.id("search-box")); // performing actions on the element searchBox.sendKeys("You have reached the Selenium Beginner's guide!"); searchBox.submit();
Interacting With Your Elements
Interacting with elements is a breeze with WebDriver’s methods. Here’s how you can click a button and type into a text field:
// clicking a button WebElement submitButton = driver.findElement(By.id("submit-button")); submitButton.click(); // typing into a text field WebElement usernameEl = driver.findElement(By.name("username")); usernameEl.sendKeys("myusername");
Execute Test
You’ve written your code, now it’s showtime! Execute your test script and watch as your browser follows your instructions. If all goes well, you’ll see your actions on the webpage. And just like that, you’ve completed your first Selenium test!
Interacting with Web Elements
Understanding the DOM
Imagine the DOM as a virtual representation of a webpage’s structure — it’s like the blueprint of a building. Each element on the page is a part of this hierarchy, and understanding it helps you interact with elements like a pro.
Using Various Locators
Locators are your tools for navigation in the DOM jungle. Let’s see how you can use different locators to find web elements:
// using ID locator WebElement usernameEl = driver.findElement(By.id("username")); // using Name locator WebElement searchBox = driver.findElement(By.name("search")); // using XPath locator WebElement signInButton = driver.findElement(By.xpath("//button[text()='Sign In']")); // using CSS Selector locator WebElement submitButton = driver.findElement(By.cssSelector(".submit-button"));
Handling Different Web Elements
Buttons, input fields, and checkboxes are just a few web elements you’ll encounter. Here’s how you can interact with them using Selenium:
// clicking a button WebElement loginButton = driver.findElement(By.id("login-button")); loginButton.click(); // typing into an input field WebElement searchEl = driver.findElement(By.name("search")); searchEl.sendKeys("Selenium rocks!"); // selecting a checkbox WebElement rememberMeChkbx = driver.findElement(By.id("remember-me")); if (!rememberMeChkbx.isSelected()) { rememberMeChkbx.click(); }
Dealing with Dynamic Web Elements
Web elements that appear or change dynamically can be a challenge, but Selenium’s got your back:
// using Explicit Wait to wait for an element to become visible WebDriverWait wait = new WebDriverWait(driver, 10); WebElement dynamicElement = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("dynamic-element"))); // using Implicit Wait to wait for a certain amount of time before throwing an exception driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // using Fluent Wait for more advanced waiting conditions Wait<WebDriver> fluentWait = new FluentWait<>(driver) .withTimeout(Duration.ofSeconds(20)) .pollingEvery(Duration.ofSeconds(5)) .ignoring(NoSuchElementException.class); dynamicElement = fluentWait.until(driver -> driver.findElement(By.id("dynamic-element")));
Future Trends and Conclusion
Emerging Trends in Automation Testing
As you embark on your Selenium journey, keeping an eye on the horizon is crucial. Automation testing is evolving faster than your favorite superhero’s costume changes. Here are a few trends you should watch out for:
AI-powered Testing: Imagine machines learning to test themselves! AI is making strides in automated testing, helping to identify patterns, predict potential issues, and even generate test scripts.
Shift-Left Testing: Testing isn’t just an afterthought anymore. Developers start testing earlier in the development cycle, catching bugs before they grow into full-blown monsters.
Microservices and API Testing: API testing is taking center stage with the rise of microservices. Seamlessly testing the interactions between services is becoming a must-know skill.
We have only scratched the surface of automation testing using Selenium in this post. If you are serious about automation testing and looking to become a professional Selenium developer, you might need a professional course from industry experts. Some of the code snippets and screen captures I’ve shared in this article are from our comprehensive course on Selenium, Java, and TestNG (w/ ChatGPT Boost): https://bit.ly/3ORVrYe
Additional Resources and References
Links to Official Selenium Documentation
Recommended Courses, and Online Communities
If you enjoyed the article and want to show your support, please:
- 👏 Give it 50 claps to boost its chances of being featured.
- 👣 Follow us on Medium for more content.
- 👥 Recommend this article to others who might benefit from it.
- 🌐 Stay connected through Facebook, LinkedIn, and Twitter.