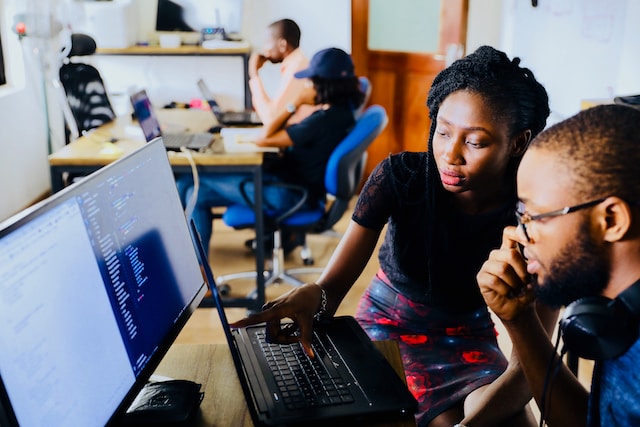
Carousels are a popular design element in web development for displaying multiple pieces of content in a limited space, creating an engaging and interactive user experience. In this comprehensive guide, we'll explore how to create dynamic and responsive carousel cards in React, allowing you to showcase images, products, or any content you desire in a visually appealing and user-friendly manner.
Introduction to React Carousel Cards
A React carousel is a UI component that allows you to display a set of content items in a horizontally or vertically scrollable container. Each content item, often referred to as a "card," can contain text, images, buttons, or any other interactive element.
React provides a flexible and efficient way to build React Bootstrap carousel cards, enabling you to create interactive and visually appealing slideshows for your website or application. In this guide, we'll walk through the step-by-step process of building a React carousel component, covering everything from setting up your project to adding interactivity and ensuring responsive design.
Setting Up Your React Project
Before diving into creating the carousel component, you'll need to set up a React project if you haven't already. You can use tools like Create React App to quickly scaffold a new React application.
npx create-react-app react-carousel-app cd react-carousel-app npm start
Once your project is set up, you're ready to start building the carousel component.
Creating the Carousel Component
Structure of a Carousel Card
Each carousel card typically consists of the following elements:
- Image or Content: The primary content to display, which can be an image, text, or a combination of both.
- Navigation Controls: Buttons or indicators to allow users to move between cards.
- Auto-Play Feature: An optional feature that automatically transitions between cards.
State Management
In React, you'll need to manage the state of your carousel component to keep track of which card is currently active. You can use the useState
hook or a state management library like Redux for more complex applications.
import React, { useState } from 'react'; function Carousel() { const [activeCard, setActiveCard] = useState(0); // ... }
Rendering Carousel Items
You can create an array of data representing your carousel cards and map through them to render each card within your Carousel
component.
import React, { useState } from 'react'; function Carousel() { const [activeCard, setActiveCard] = useState(0); const carouselData = [ // Card 1 data // Card 2 data // ... ]; return ( <div className="carousel"> {carouselData.map((card, index) => ( <div key={index} className={`carousel-card ${index === activeCard ? 'active' : ''}`}> {/* Render card content here */} </div> ))} </div> ); }
Styling Your Carousel
CSS Frameworks
You can leverage CSS frameworks like Bootstrap, Material-UI, or Semantic UI to style your carousel quickly. These frameworks often provide pre-designed components and styles that can be customized to match your project's look and feel.
Custom Styling
If you prefer a more custom approach, you can style your carousel using CSS or preprocessors like Sass or LESS. Consider CSS-in-JS libraries like Styled Components for a more component-based styling approach.
import styled from 'styled-components'; const CarouselCard = styled.div` /* Define your styles here */ `; function Carousel() { // ... return ( <div className="carousel"> {carouselData.map((card, index) => ( <CarouselCard key={index} className={`carousel-card ${index === activeCard ? 'active' : ''}`}> {/* Render card content here */} </CarouselCard> ))} </div> ); }
Adding Interactivity
A well-designed carousel is interactive and allows users to navigate between cards and control the slideshow.
Navigation Controls
You can add navigation controls such as "Previous" and "Next" buttons or slide indicators to let users move between cards.
function Carousel() { // ... return ( <div className="carousel"> {carouselData.map((card, index) => ( <CarouselCard key={index} className={`carousel-card ${index === activeCard ? 'active' : ''}`}> {/* Render card content here */} </CarouselCard> ))} <button onClick={() => setActiveCard(activeCard - 1)}>< Previous</button> <button onClick={() => setActiveCard(activeCard + 1)}>Next ></button> </div> ); }
Auto-Play Feature
To make your carousel even more engaging, you can implement an auto-play feature that automatically transitions between cards at a set interval.
import { useEffect } from 'react'; function Carousel() { // ... // Auto-play settings const autoPlayInterval = 5000; // 5 seconds useEffect(() => { const interval = setInterval(() => { // Logic to advance to the next card setActiveCard((prev) => (prev + 1) % carouselData.length); }, autoPlayInterval); return () => clearInterval(interval); }, []); // ... }
Responsive Design
Creating a responsive carousel ensures that it adapts to various screen sizes and orientations. You can achieve this by using CSS media queries to adjust the carousel's layout and card sizes based on the viewport dimensions.
/* Example of a media query for a mobile-friendly carousel */ @media (max-width: 768px) { .carousel-card { /* Adjust card size or layout for smaller screens */ } }
Optimizing for Performance
Optimizing your carousel for performance is crucial, especially if you have a large number of cards or media-heavy content. Consider implementing lazy loading for images and minimizing re-renders by using React's React.memo
or shouldComponentUpdate
for class-based components.
Common Challenges and Solutions
While building a React carousel, you may encounter challenges such as touch support for mobile devices, accessibility considerations, and ensuring smooth transitions. These challenges can be addressed with libraries like react-swipeable
for touch events and proper ARIA attributes for accessibility.
Testing Your React Carousel
Thoroughly testing your carousel component is essential to ensure it works as expected. You can use testing libraries like React Testing Library or Enzyme to write unit and integration tests.
Deployment and Best Practices
When deploying your React application with a carousel, consider using a content delivery network (CDN) for media assets to improve load times. Additionally, follow best practices for web performance and SEO to ensure your carousel is accessible and performs well in search engine rankings.
Conclusion
Building an interactive React carousel is a rewarding endeavor that can greatly enhance the user experience of your web application. By following the steps outlined in this guide, you can create a dynamic and responsive carousel that effectively showcases your content.
Partnering with CronJ means gaining access to a dedicated team of hire react.js developers who are committed to delivering high-quality solutions. Whether you're a startup looking to build your first web app or an enterprise seeking to enhance your online presence, CronJ has the knowledge and resources to turn your vision into reality.
Remember to consider factors like styling, interactivity, responsiveness, and performance optimization to ensure your carousel not only looks great but also functions smoothly across various devices and screen sizes. With the knowledge and skills gained from this guide, you're well-equipped to embark on your journey of creating captivating carousel experiences in your React projects. Happy coding!