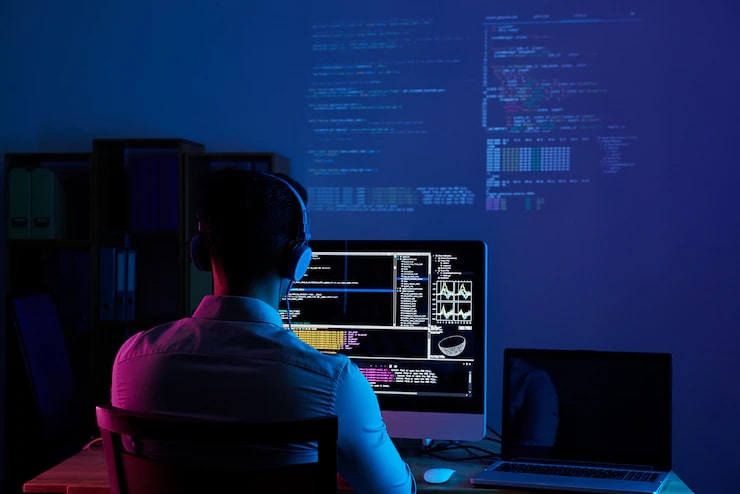
This article gives you a comprehensive guide on smart contract development and deployment on the NEAR platform.
NEAR Protocol is a decentralised blockchain app development platform that has been dubbed the “Ethereum killer.” It attempts to make the process of creating dApps simpler and more user-friendly for developers. Users can use its native token, NEAR, to pay for storage and transaction costs on the NEAR platform.
The best illustration of a third-generation digital ledger is the NEAR Protocol. It concentrates on addressing scalability problems. It encourages the network to create and introduce dApps into the ecosystem to support decentralised finance.
The two distinct parts of the NEAR Protocol
- Native token named NEAR
- Smart Contracts
They manage the on-chain storage. Modification of data.
Interactions with Smart Contract-(s):-
We are able to communicate with both the contracts we deploy and the contracts that others install.
How to create a Smart Contract on NEAR Protocol
https://wallet.testnet.near.org and select the ‘create account’ option. Then, insert the testnet account name that you wish to use
Pre-requisites
- Rust toolchain
- A NEAR account
- NEAR command-line interface (near-cli)
Set up requirements
Using the Rust environment create a NEAR account and then install near-cli.
- Install Rust toolchain
- Install the Rustup
Install the Rustup:
curl — proto ‘=https’ — tlsv1.2 -sSf https://sh.rustup.rs | sh
Configure the current shell
Insert the following command into the configuration of your current shell:
source $HOME/.cargo/env
Add the wasm target to the blockchain network
rustup target add wasm32-unknown-unknown
Repository Creation
cargo new calculator
cd calculator
Editing Cargo.toml
[package]
name = “rust-counter-tutorial”
version = “0.1.0”
authors = [“NEAR Inc <[email protected]>”]
edition = “2018”
[lib]
crate-type = [“cdylib”, “rlib”]
[dependencies]
near-sdk = “3.1.0”
[profile.release]
codegen-units = 1
# Tell `rustc` to optimize for small code size.
opt-level = “z”
lto = true
debug = false
panic = “abort”
# Opt into extra safety checks on arithmetic operations https://stackoverflow.com/a/64136471/249801
overflow-checks = true
Create Lib.rs
use near_sdk::borsh::{self, BorshDeserialize, BorshSerialize};
use near_sdk::{env, near_bindgen};
near_sdk::setup_alloc!();
#[near_bindgen]
#[derive(Default, BorshDeserialize, BorshSerialize)]
pub struct Calculator {
x: i64,
y: i64,
}
#[near_bindgen]
impl Calculator {
pub fn get_numbers(&self) -> (i64, i64) {
(self.x, self.y)
}
pub fn add_numbers(&mut self) -> i64 {
let add = self.x + self.y;
let log_message = format!(“Addition of numbers {} + {} = {}”, self.x, self.y, add);
env::log(log_message.as_bytes());
add
}
pub fn sub_numbers(&mut self) -> i64 {
let sub = self.x — self.y;
let log_message = format!(“Subtraction of numbers {} — {} = {}”, self.x, self.y, sub);
env::log(log_message.as_bytes());
sub
}
pub fn mul_numbers(&mut self) -> i64 {
let mul = self.x * self.y;
let log_message = format!(
“Multiplication of numbers {} * {} = {}”,
self.x, self.y, mul
);
env::log(log_message.as_bytes());
mul
}
pub fn div_numbers(&mut self) -> i64 {
let div = self.x / self.y;
let log_message = format!(“Division of numbers {} / {} = {}”, self.x, self.y, div);
env::log(log_message.as_bytes());
div
}
}
// use the attribute below for unit tests
#[cfg(test)]
mod tests {
use near_sdk::MockedBlockchain;
use near_sdk::{testing_env, VMContext};
use crate::Calculator;
fn get_context(input: Vec<u8>, is_view: bool) -> VMContext {
VMContext {
current_account_id: “alice.testnet”.to_string(),
signer_account_id: “robert.testnet”.to_string(),
signer_account_pk: vec![0, 1, 2],
predecessor_account_id: “jane.testnet”.to_string(),
input,
block_index: 0,
block_timestamp: 0,
account_balance: 0,
account_locked_balance: 0,
storage_usage: 0,
attached_deposit: 0,
prepaid_gas: 10u64.pow(18),
random_seed: vec![0, 1, 2],
is_view,
output_data_receivers: vec![],
epoch_height: 19,
}
}
// mark individual unit tests with #[test] for them to be registered and fired
#[test]
fn addition() {
// set up the mock context into the testing environment
let context = get_context(vec![], false);
testing_env!(context);
// instantiate a contract variable with the counter at zero
let mut contract = Calculator { x: 20, y: 10 };
let num = contract.add_numbers();
println!(“Value after addition: {}”, num);
// confirm that we received 1 when calling get_num
assert_eq!(30, num);
}
#[test]
fn subtraction() {
// set up the mock context into the testing environment
let context = get_context(vec![], false);
testing_env!(context);
// instantiate a contract variable with the counter at zero
let mut contract = Calculator { x: 20, y: 10 };
let num = contract.sub_numbers();
println!(“Value after subtraction: {}”, num);
// confirm that we received 1 when calling get_num
assert_eq!(10, num);
}
#[test]
fn multiplication() {
// set up the mock context into the testing environment
let context = get_context(vec![], false);
testing_env!(context);
// instantiate a contract variable with the counter at zero
let mut contract = Calculator { x: 20, y: 10 };
let num = contract.mul_numbers();
println!(“Value after multiplication: {}”, num);
// confirm that we received 1 when calling get_num
assert_eq!(200, num);
}
#[test]
fn division() {
// set up the mock context into the testing environment
let context = get_context(vec![], false);
testing_env!(context);
// instantiate a contract variable with the counter at zero
let mut contract = Calculator { x: 20, y: 10 };
let num = contract.div_numbers();
println!(“Value after division: {}”, num);
// confirm that we received 1 when calling get_num
assert_eq!(2, num);
}
}
Test the code
Test the smart contract code via cargo,
cargo test
You will then receive output in the form of:
Compiling rust-counter-tutorial v0.1.0 (/home/yogesh/Blogs/rust-counter)
Finished test [unoptimized + debuginfo] target(s) in 1.57s
Running unittests src/lib.rs (target/debug/deps/rust_counter_tutorial-7e3850288c4d6416)
running 4 tests
test tests::division … ok
test tests::addition … ok
test tests::multiplication … ok
test tests::subtraction … ok
test result: ok. 4 passed; 0 failed; 0 ignored; 0 measured; 0 filtered out; finished in 0.00s
Doc-tests rust-counter-tutorial
running 0 tests
test result: ok. 0 passed; 0 failed; 0 ignored; 0 measured; 0 filtered out; finished in 0.00s
Compile the code
cargo build — target wasm32-unknown-unknown — release
Deploying smart contracts
You will deploy it using the near-cli testnet of your NEAR account.
near login
near deploy — wasmFile target/wasm32-unknown-unknown/release/rust_counter_tutorial.wasm — accountId YOUR_ACCOUNT_HERE
You will then receive output in the form of:
To see the transaction in the transaction explorer, please open this url in your browser
https://explorer.testnet.near.org/transactions/CqEh5tsh9Vo827DkN4EurECjK1ft8pRdkY2hhCYN9W8i
Done deploying to yogeshsha.testnet
If you want more information on how to get started with NEAR blockchain-based dApp development, you may connect with our Smart Contract Developers.